Between Coffee Breaks and Console Logs
Where lifestyle, late night learning, and logic errors meet and no one asks if you belong here.
Some learners sit down with a clean desk and a quiet hour. Others squeeze in 15 minutes between reheating leftovers and reloading a stuck browser tab. A Girl Among Geeks was built for the second kind.
It’s a space for the ones who don’t wait for perfect conditions who pick at the problem during lunch breaks, late nights, or right after the baby’s finally asleep. Where headphones are half-working, notes are scattered, and half the error logs don’t even make sense. Yet somehow, progress happens.
That’s why nothing here feels out of reach. The posts are direct, written in plain language, and tackle the small things that block real people: a container that won’t build, a dock icon that won’t stay, a ZIP driver that just won’t unpack.
It’s not about perfection it’s about momentum. And if you’ve got just a little bit of that today, you’re in the right place.
Even five minutes of clarity is still progress.


Not Just Syntax – It’s Self-Taught Strength
From curly braces to confidence every article carries a little more than just answers.
Learning to code alone is more than just typing things until they work. It’s sitting with errors no one prepared you for. It’s copying something that worked once and wondering why it doesn’t anymore. It’s reading five explanations and still not feeling sure.
That’s the part A Girl Among Geeks leans into the hesitation before you press enter, the quiet frustration that builds, and the steady decision to keep learning anyway.
The posts here aren’t written to impress. They’re written to explain. Every guide is built to clear fog, not just fix bugs whether it’s breaking down SQL joins, catching silent logic errors, or finally understanding why your grid layout won’t behave.
What matters most isn’t just finding the answer. It’s knowing how to handle the next one.
And if that confidence hasn’t shown up yet, that’s fine. Keep going. It’s building underneath everything you’re figuring out.
Clear answers build confidence one fix at a time.
Real talk from the keyboard of Barbara Hernandez
I’m Barbara a self-taught techie who learned to navigate error messages before I learned to write elegant code. I didn’t step into programming with a perfect plan or a computer science degree. I just got tired of not knowing how things worked… and decided to fix that, one late-night search at a time.
That’s how A Girl Among Geeks came to life not as a brand, but as a place for honest, no shame answers to questions we all have. You’ll find me writing about everything from container glitches and database quirks to random things like “Why won’t my app stick to the Mac dock?”
This site isn’t here to impress it’s here to help. And if you’ve ever felt too embarrassed to ask something you “should already know,” then this space was built for you.
Let’s break things down, one bug at a time.

What Have We Covered In This Blog?
Figuring It Out, One Error at a Time
We tackle the real blockers: vague errors, build failures, or tools that suddenly misbehave. With every guide, we focus on practical clarity walking you through what broke, why it did, and how to fix it for good.
Code That Works in Real Life
This isn’t about perfect code it’s about code that survives real projects. We explain the quirks, fixes, and setups that help you build usable logic, clean structure, and stable results without missing the “why” behind what works.
Tools You Didn’t Know You Needed
Some problems start before the code: mismatched environments, system conflicts, or hidden dependencies. We cover the overlooked layers that affect your work and show you how to set things up right from the ground up.
The Gaps Most Guides Skip
When help documents jump steps, we fill them in. From context that was never explained to questions never asked, this space walks you through what others assume you already know without the guesswork or gatekeeping.
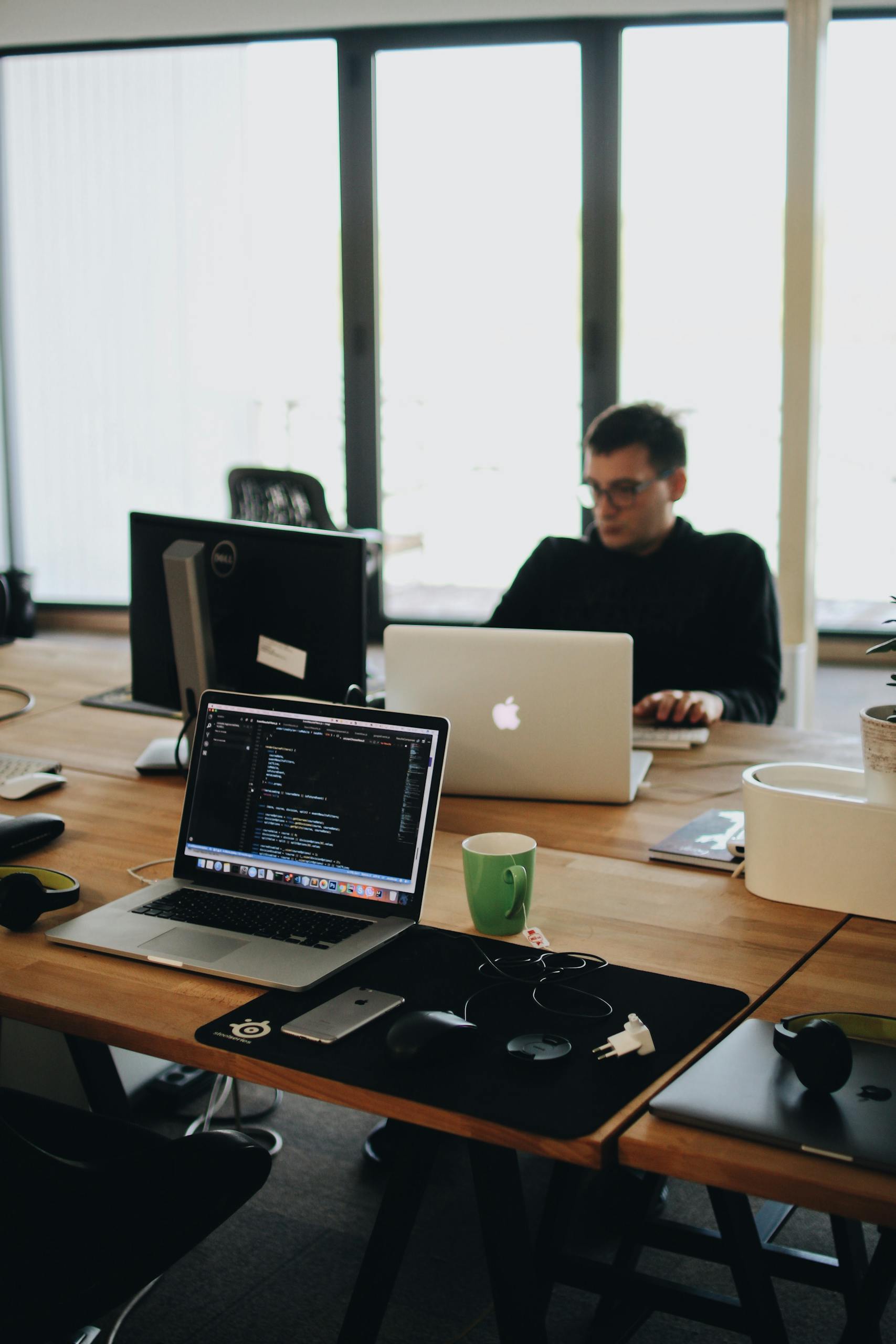
From “What Went Wrong?” to “Now I Get It”
Everyone hits a wall. At Freak Learn, we help you push through it.
This site is about that turning point when a vague error becomes an “aha” moment. We know what it’s like to follow tutorials that skip steps, or documentation that assumes you know everything already. That’s why every guide here is written with clarity and approachability in mind.
We explain not just what worked, but why it worked because that’s how real learning sticks. From subtle syntax issues and dependency conflicts to OS quirks and query bugs, Freak Learn is designed to demystify the mess and show you what’s really going on under the hood.
We’re not here to overwhelm you with options or show off expertise. We’re here to simplify the hard stuff so you can move forward with confidence.
Understanding starts when frustration ends.
Why This Space Feels Different
Unlike sites that skim the surface or assume you already “get it,” A Girl Among Geeks slows down, digs deeper, and speaks plainly. It’s built for learners who want clarity without condescension, and solutions that match real-life chaos. No filler, no ego just answers that actually help you move forward.
Categories
Explore scripting essentials, DOM behavior, and everyday logic challenges through hands-on explanations that make JavaScript easier to use & understand across different development needs .
Get practical guidance for writing, compiling, and managing Java code including handling errors, and understanding how Java fits into real-world application development environments.
Unpack syntax, execution flow, and hidden quirks of Go. Whether you’re building from scratch or revisiting core functions, find clarity on handling concurrency, packages, and efficient structure.
Learn how to manage containers, solve image conflicts. This section helps demystify Docker setups & encourages confidence through real fixes, not just repeated commands.
Tame layout troubles, fix spacing mishaps, and gain a working understanding of how CSS actually behaves across browsers including quick wins for broken grids and stubborn elements.
From faulty connections to upgrade advice, this section addresses everyday hardware concerns developers face, setup, and smarter decisions when something just won’t work.
Get past vague errors and tangled plugins. This section covers practical fixes, tweaks, and answers that make managing WordPress feel less overwhelming and far more manageable for daily use.
Make sense of Ubuntu environments from terminal basics to package installation errors with guides focused on stability, fixes, and comfort for those transitioning into Linux systems.
Clarify how types work, where declarations break, and why the compiler’s yelling again. This section helps demystify TypeScript in ways that support growth, not just project builds.
A space for the head scratchers & the mystery bugs. Find logic-first explanations and workarounds that speak to real learners navigating confusing behavior in code.
We rephrase the answers you almost understood. Here, popular but unclear Stack Overflow threads get rewritten with simplicity, context, and patience for readers still learning their way.
Break down real database problems and questions into digestible logic. This category helps you write, & debug SQL with confidence even when syntax or results don’t make sense.
Walk through key Python concepts with clarity. Perfect for those building confidence while tackling exceptions, & practical scripts that actually run.
Understand runtime behavior, resolve strange module issues, and connect the dots between server logic. Node becomes less mysterious when explained in context, not just syntax.
Cover permissions, processes, and odd behaviors that trip up new users. This section builds Linux confidence by focusing on what goes wrong and how to fix it fast.
Learn to live inside the terminal, track down file paths, and recover from input mistakes. This category makes navigating Linux feel less cryptic and far more approachable for beginners.
From deployment issues to container orchestration confusion, this section simplifies Kubernetes by unpacking what’s happening behind the scenes so you can solve problems without drowning in terminology.
Handle structure errors, parse failures, and formatting issues that block your flow. These guides help make JSON behave, while also helping you actually understand what’s breaking and why.